In Sitecore we can use available Send Email Submit Action to send the email, In the below screen shot we can see the available option to configure the Send Email action for Submit button.
So far so good, now let’s take an example where we want to send an email with the customized message body, how to go there? We have solution like using MailMessage class and setting message properties like Subject, Body, from address etc.. and then create SMTP client to send email.
We can also leverage SendEmail submit action and update the message body to send custom data, this would be useful for such requirements like where we want to send some activation link, data to our end users for confirmation.
Let’s see how we can use second option and send customized message body.
Here are the steps required:
- Create a class and inherit that class with Sitecore Forms SubmitActions SendEmail class.
1 | public class SendCustomEmail : Sitecore.ExperienceForms.Mvc.Processing.SubmitActions.SendEmail |
- Initialize a new instance of the class you created above.
1 2 3 4 | public SendCustomEmail (ISubmitActionData submitActionData) : base(submitActionData) { } |
- Next step is to override Execute method with SendEmailData/FormSubmitContext parameters, set the SendEmaiData Body property using custom value and perform other business rule(s) as per project/business requirements.
1 2 3 4 5 6 7 8 9 10 11 12 13 | protected override bool Execute(SendEmailData data, FormSubmitContext formSubmitContext) { Assert.ArgumentNotNull(formSubmitContext, nameof(formSubmitContext)); if (!formSubmitContext.HasErrors) { // Process formSubmitContext here... var emailBody = "Custom Email Message"; // Set custom email body message here... data.Body = data.Body.Replace("#email#", emailBody); } return true; } |
- In the above step we have set the Body property of SendEmailData class and replaced that value with a placeholder text which is set as part of Sitecore form we created.

- Next step is to call base class Execute method and you are done.
1 | base.Execute(data, formSubmitContext); |
- After this we need to create a new submit action in Sitecore and configure the required fields like Model Type, Error Message and Editor.
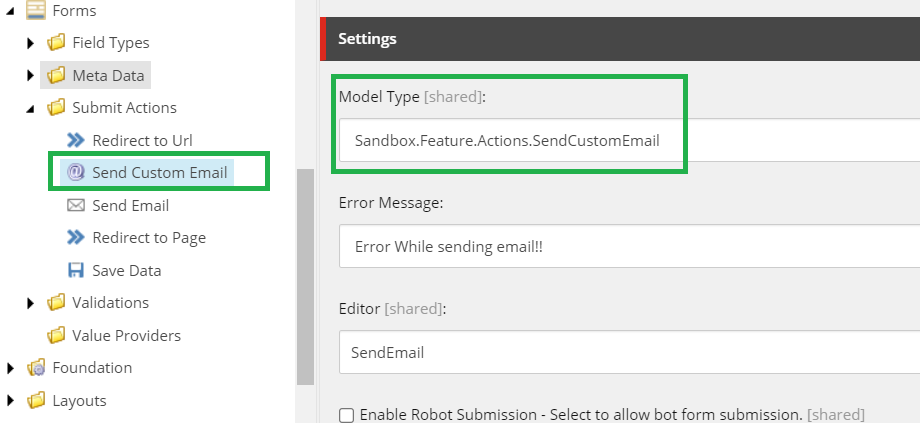
- Once this is done you just need to select the newly created submit action as part of the Submit button and you are good to go.
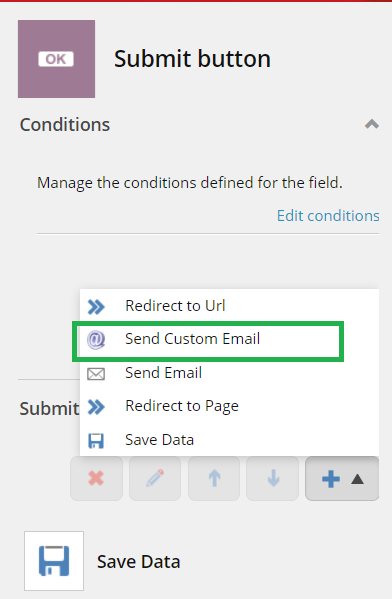
Here is the full code for ref.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | // <copyright file="SendCustomEmail.cs" company="Sandbox"> // Copyright (c) Sandbox. All rights reserved. // </copyright> namespace Sandbox.Feature.Actions { using Sitecore.Diagnostics; using Sitecore.ExperienceForms.Models; using Sitecore.ExperienceForms.Mvc.Models.SubmitActions; using Sitecore.ExperienceForms.Processing; public class SendCustomEmail : Sitecore.ExperienceForms.Mvc.Processing.SubmitActions.SendEmail { /// <summary> /// Initializes a new instance of the <see cref="SendCustomEmail"/> class. /// </summary> /// <param name="submitActionData">submitActionData.</param> public SendCustomEmail(ISubmitActionData submitActionData) : base(submitActionData) { } /// <summary> /// Executes the action with the specified <paramref name="data" />. /// </summary> /// <param name="data">The data.</param> /// <param name="formSubmitContext">The form submit context.</param> /// <returns><c>true</c> if the action is executed correctly; otherwise <c>false</c>.</returns> protected override bool Execute(SendEmailData data, FormSubmitContext formSubmitContext) { Assert.ArgumentNotNull(formSubmitContext, nameof(formSubmitContext)); if (!formSubmitContext.HasErrors) { // Process formSubmitContext here... var emailBody = "Custom Email Message"; // Set custom email body message here... data.Body = data.Body.Replace("#email#", emailBody); base.Execute(data, formSubmitContext); } return true; } } } |
Hope this helps!
To know about Sitecore forms, please refer Sitecore documentation for details- https://doc.sitecore.com/en/users/90/sitecore-experience-platform/sitecore-forms.html
Also If you want to checkout my latest Sitecore videos, you can do so here on Youtube- https://www.youtube.com/c/ankitjoshi2409
Happy learning
Comments
Post a Comment